Hello folks. I was trying to figure out how to setup express and mongodb with container and depended services. There were lot of tutorials but there were missing things here and there. I had to go through multiple sites to get a simple and short app running.
So, here is a post describing how to set it up!
I am assuming you have docker, docker-compose setup already done. I am using VSCode as editor to do all my stuff which is way easier now with the new releases that Microsoft has done. I highly recommend to switch to VSCode.
Now, I want to create a simple express app using a mongo connection with no business logic for now. Hence my package.json and index.js is very simple.
db.js:
Plain and simple with just DB URL. the catch here is the “mongo” service name. Docker containers will use internal DNS to resolve this to the the mongo container and do the magic.
index.js
This is a simple main application file for express. Nothing fancy here. Just listens to DB and puts out a json for the homepage.
package.json
Nothing here just my dependencies for express and mongo.

On the vscode project, we can automate the generation of docker files via plugin but lets skip that for now and stick to basics. We have to create 3 files – Dockerfile, docker-compose.yml and .dockerignore.
Dockerfile
This is a simple docker file for express application stating docker to pull the node version and copy the code to server and open 3000 port to outside because that is the port we run the application.
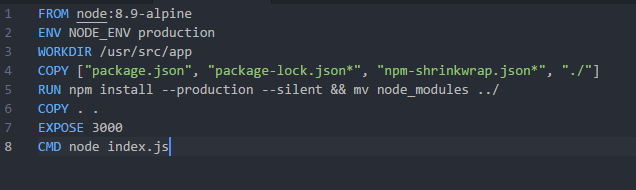
.dockerignore
This is also pretty standard which tells docker-compose to ignore few things like gitignore.
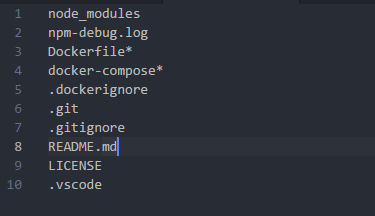
docker-compose.yml
Now, this is the file where we have to look for the magic.
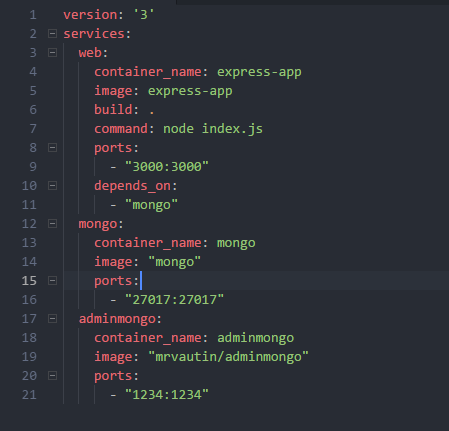
Few things to look for:
- this sets up the main application which points to the root directory with “Dockerfile” and exposing container 3000 port to outside port
- Main application depends on mongo service which we have defined next. This just tells docker to define the order in which the containers should be started. Hence it will start “mongo” before “express-app”. [Note: there is an issue here that I will discuss down the post]
- “mongo” service name is used. This is same as the db.js file reference to the DNS. This is how docker will connect two containers.
- “adminmongo” is just an application to browse mongo databases via web. I highly recommend to use it.
That’s it we can now build and run the application.
Below command to build and run
-
docker-compose build
-
docker-compose up -d
Now you will notice that your express-app sometime don’t connect to Mongo. This is because docker only controls the start order of services not the ready state. Hence once docker has issued a start command to mongo, it will issue start of express and express app will throw error for mongo not being up.
Now, to resolve this there are 2 ways:
- Just restart the express-app container using command “docker restart <CONTAINER_ID>
- Do some scripting in “command” section of express-app to either poll for mongo to be up or wait for predefined time to start the app.
In my view, for development, it is ok to restart the container only but best approach for production grade applications is to have tests and scripts to ensure that all dependencies are up!
I would expect docker to have a check for ready state as well may be via some plugin. Please comment if you know of any!
Drop a like or share and happy coding!